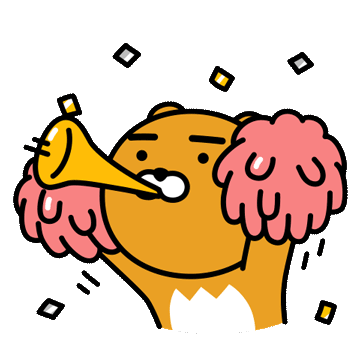
새해 복 많이 받으세요들~
미니 프로젝트 주차가 끝나고 드디어 본격적인 C# 종합문법 주차가 시작되었다.
문법 첫 주차는 팀 과제가 아닌 개인과제가 미션으로 주어졌다. 과제내용은
스파르타 Text 던전 만들기
콘솔로 작업하는건 굉장히 오랜만이다. 마침 깃허브로 제출이다보니 깃허브에대한 숙련도도 함께 쌓을 수 있어서 좋구만?
요구사항에는 4가지 필수사항과 8가지 선택사항이 있다.
그 중 오늘은 2가지 필수사항을 구현해보자.
1. 게임 시작 화면
2. 상태보기
이 두가지를 구현해보도록 하자.
먼저 비쥬얼스튜디오를 켜서 새로운 프로젝트를 생성하자. (2022버전을 사용했다.)
프로젝트를 만들었으니 이제 게임을 만들어 보자.
1. 게임 시작 화면
먼저 프로그램을 실행 했을 때 1. 상태 / 2. 인벤토리 / 3. 상점 으로 연결되는 마을(?)을 만들어보자.
static void Main(string[] args)
{
// while 문으로 게임을 종료하지 않는이상 계속 실행되도록 해준다.
while (true)
{
Console.WriteLine("※※※17초 던전 마을에 온 것을 환영합니다.※※※");
Console.WriteLine("※※※원하시는 행동을 선택하세요.※※※\n");
Console.WriteLine("(1) : [상태]\n(2) : [인벤토리]\n(3) : [상점]\n");
// string 형식의 input 변수에 콘솔에 입력하는 값이 담기도록한다.
string? input = Console.ReadLine();
// switch 문으로 입력된 input 값에 따라 실행되는 명령이 달라진다.
switch (input)
{
case "1":
Console.Clear();
Status();
break;
case "2":
Console.Clear();
// 인벤토리
break;
case "3":
Console.Clear();
// 상점
break;
case "4":
Console.ForegroundColor = ConsoleColor.DarkBlue;
Console.WriteLine("※※※게임을 종료합니다※※※");
Console.ResetColor();
return;
default:
Console.Clear();
Console.ForegroundColor = ConsoleColor.Red;
Console.WriteLine("!!!잘못된 입력입니다!!!");
Console.ResetColor();
break;
}
}
}
}
콘솔창에 '4'를 입력하면 게임을 종료한다는 문구와 함께 return 으로 while문을 종료시키고 프로그램이 종료된다.
1, 2, 3, 4 이외의 값을 입력하면 잘못된 입력이라는 경고문구와 함께 while문을 1회 루프 해 입력단계로 다시 돌아온다.
2. 상태보기
콘솔창에 '1'을 입력하면 상태창을 보여주도록 한다. ('2', '3' 은 추후 구현.)
static void Status(ref Player player)
{
while (true)
{
Console.ForegroundColor = ConsoleColor.Green;
Console.WriteLine("[상태 창]\n");
Console.ResetColor();
Console.WriteLine("※※※원하시는 행동을 선택하세요.※※※\n");
Console.WriteLine("(1) : [인벤토리]\n(2) : [상점]\n(3) : [마을로 돌아가기]\n");
Console.WriteLine("원하시는 행동을 입력해주세요.");
string? input = Console.ReadLine();
switch (input)
{
case "1":
Console.Clear();
// 인벤토리
return;
case "2":
Console.Clear();
// 상점
return;
case "3":
Console.Clear();
return;
default:
Console.Clear();
Console.ForegroundColor = ConsoleColor.Red;
Console.WriteLine("!!!잘못된 입력입니다!!!");
Console.ResetColor();
break;
}
}
}
하지만 플레이어 정보가 없기 때문에 상태 창에 보여줄 정보가 없다.
지금부터 플레이어를 생성하고 상태 창에 플레이어 정보를 출력하자.
public enum PlayerClass
{
Worrior,
Archer,
Magic,
Thief,
}
internal class Program
{
struct Player
{
public int lv;
public string name;
public PlayerClass m_class;
public int atk;
public int def;
public int hp;
public int gold;
}
static void CharacterCreate(ref Player player)
{
player.lv = 1;
player.name = "십칠조";
player.m_class = PlayerClass.Worrior;
player.atk = 10;
player.def = 5;
player.hp = 100;
player.gold = 1500;
}
static void Main(string[] args)
{
Player player = new Player();
CharacterCreate(ref player);
SelectAction(ref player);
}
}
}
enum 열거형으로 플레이어의 직업을 미리 여러개 만들어두자.
그리고 구조체로 플레이어 정보를 담아 메인함수에서 플레이어를 새로 만들도록 하고,
CharacterCreate 함수에 ref Player 를 넘겨줘 정보를 할당하도록 하자.
ref 로 넘기는 이유는 구조체는 값 형식이기 때문에 일반 player를 넘기게 되면
player의 복사본을 받게되어 원본 player에 영향이 없다.
player의 정보는 당장은 정해놓은 정보로 할당해주자.
Main 함수에서 SelectAction 함수에 마을에서의 코드를 넣어주고 마찬가지로 ref 로 player를 넘겨주자.
namespace RtanTextDungeon
{
internal class Program
{
static void SelectAction(ref Player player)
{
// 코드는 위와 동일
}
static void Status(ref Player player)
{
while (true)
{
Console.ForegroundColor = ConsoleColor.Green;
Console.WriteLine("[상태 창]\n");
Console.ResetColor();
Console.WriteLine("---------------------------------\n");
Console.WriteLine($"Lv. {player.lv.ToString("00")}\n\n" +
$"{player.name} ({player.m_class})\n" +
$"공격력 : {player.atk}\n" +
$"방어력 : {player.def}\n" +
$"체 력 : {player.hp}\n" +
$"Gold : {player.gold}\n");
Console.WriteLine("---------------------------------\n");
// 코드는 위와 동일
}
}
}
static void Main(string[] args)
{
Player player = new Player();
CharacterCreate(ref player);
SelectAction(ref player);
}
}
}
SelectAction 과 Status 함수 둘 다 player 정보를 ref 로 받고 Status 함수 내에서 player 정보를 출력해주자.
'스파르타 내배캠' 카테고리의 다른 글
스파르타 내배캠 Unity 3기 8일차 (1) | 2024.01.03 |
---|---|
스파르타 내배캠 Unity 3기 7일차 (1) | 2024.01.02 |
스파르타 내배캠 Unity 3기 5일차 (0) | 2023.12.28 |
스파르타 내배캠 Unity 3기 4일차 (1) | 2023.12.27 |
스파르타 내배캠 Unity 3기 3일차 (2) | 2023.12.26 |